数组
数组的优点和缺点,并且要理解为什么。
第一:空间存储上,内存地址是连续的。
第二:每个元素占用的空间大小相同。
第三:知道首元素的内存地址。
第四:通过下标可以计算出偏移量。通过一个数学表达式,就可以快速计算出某个下标位置上元素的内存地址,直接通过内存地址定位,效率非常高。
优点:检索效率高。
缺点:随机增删效率较低,数组无法存储大数据量。
注意:数组最后一个元素的增删效率不受影响。
一维数组
一维数组的静态初始化和动态初始化
静态初始化:
1 2
| int[] arr = {1,2,3,4}; Object[] objs = {new Object(), new Object(), new Object()};
|
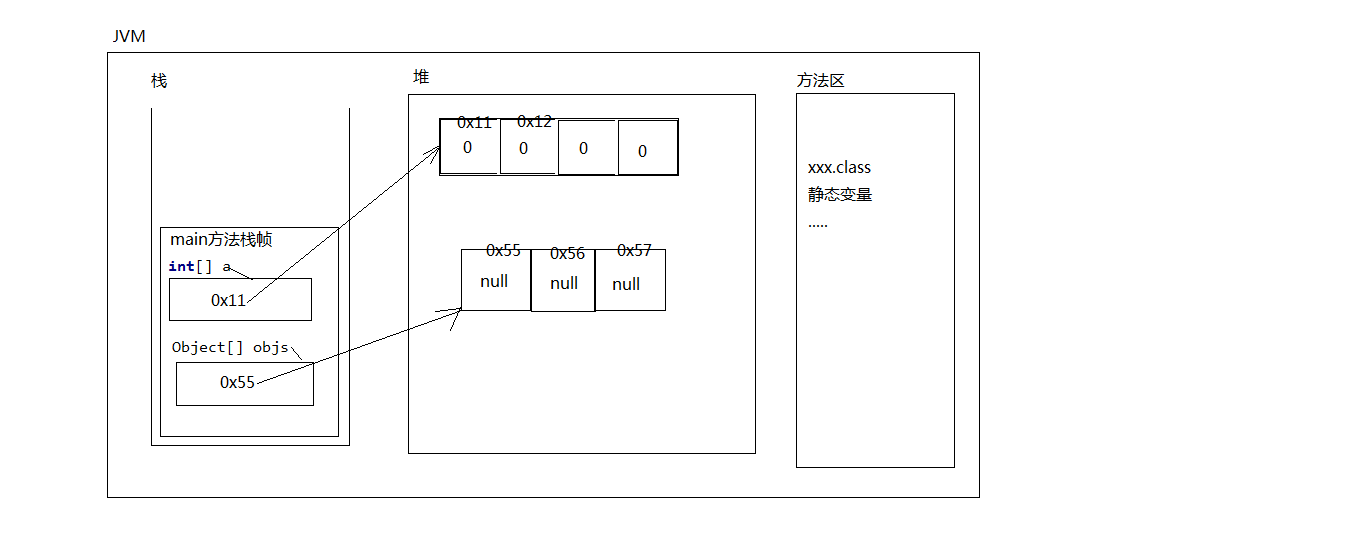
动态初始化:
1 2
| int[] arr = new int[4]; Object[] objs = new Object[4];
|
一维数组的遍历
for(int i = 0; i < arr.length; i++){
System.out.println(arr[i]);
}
一维数组储存对象的调用 多态的调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109
| package com.bjpowernode.javase.array;
public class ArrayTest07 { public static void main(String[] args) {
int[] a = {100, 200, 300}; System.out.println(a[1]);
int[] array = {1,2,3}; for (int i = 0; i < array.length; i++) {
System.out.println(array[i]); }
Animal a1 = new Animal(); Animal a2 = new Animal(); Animal[] animals = {a1, a2};
for (int i = 0; i < animals.length; i++) {
animals[i].move(); }
Animal[] ans = new Animal[2]; ans[0] = new Animal();
ans[1] = new Cat();
Cat c = new Cat(); Bird b = new Bird(); Animal[] anis = {c, b};
for (int i = 0; i < anis.length; i++){
if(anis[i] instanceof Cat){ Cat cat = (Cat)anis[i]; cat.catchMouse(); }else if(anis[i] instanceof Bird){ Bird bird = (Bird)anis[i]; bird.sing(); } }
} }
class Animal{ public void move(){ System.out.println("Animal move..."); } }
class Product{
}
class Cat extends Animal { public void move(){ System.out.println("猫在走猫步!"); } public void catchMouse(){ System.out.println("猫抓老鼠!"); } }
class Bird extends Animal { public void move(){ System.out.println("Bird Fly!!!"); } public void sing(){ System.out.println("鸟儿在歌唱!!!"); } }
|
二维数组
二维数组的静态初始化和动态初始化
静态初始化:
1 2 3 4
| int[][] arr = {{1,2,34},{54,4,34,3},{2,34,4,5}}; Object[][] arr = {{new Object(),new Object()}, {new Object(),new Object()}, {new Object(),new Object(),new Object()}};
|
动态初始化:
1 2 3 4 5 6
| int[][] arr = new int[3][4]; Object[][] arr = new Object[4][4]; Animal[][] arr = new Animal[3][4];
Person[][] arr = new Person[2][2]; ....
|
二维数组的遍历
for(int i = 0; i < arr.length; i++){ // 外层for循环负责遍历外面的一维数组。
// 里面这个for循环负责遍历二维数组里面的一维数组。
for(int j = 0; j < arr[i].length; j++){
System.out.print(arr[i][j]);
}
// 换行。
System.out.println();
}
main方法上“String[] args”参数的使用
(非重点,了解一下,以后一般都是有界面的,用户可以在界面上输入用户名和密码等参数信息。)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
|
public class ArrayTest06 { public static void main(String[] args) { if(args.length != 2){ System.out.println("使用该系统时请输入程序参数,参数中包括用户名和密码信息,例如:zhangsan 123"); return; }
String username = args[0]; String password = args[1];
if("admin".equals(username) && "123".equals(password)){ System.out.println("登录成功,欢迎[" + username + "]回来"); System.out.println("您可以继续使用该系统...."); }else{ System.out.println("验证失败,用户名不存在或者密码错误!"); } } }
|
数组的拷贝:System.arraycopy()方法的使用
数组有一个特点:长度一旦确定,不可变。 所以数组长度不够的时候,需要扩容,扩容的机制是:新建一个大数组,将小数组中的数据拷贝到大数组,然后小数组对象被垃圾回收。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
|
public class ArrayTest08 { public static void main(String[] args) {
int[] src = {1, 11, 22, 3, 4};
int[] dest = new int[20];
System.arraycopy(src, 0, dest, 0, src.length); for (int i = 0; i < dest.length; i++) { System.out.println(dest[i]); }
String[] strs = {"hello", "world!", "study", "java", "oracle", "mysql", "jdbc"}; String[] newStrs = new String[20]; System.arraycopy(strs, 0, newStrs, 0, strs.length); for (int i = 0; i < newStrs.length; i++) { System.out.println(newStrs[i]); }
System.out.println("================================"); Object[] objs = {new Object(), new Object(), new Object()}; Object[] newObjs = new Object[5]; System.arraycopy(objs, 0, newObjs, 0, objs.length); for (int i = 0; i < newObjs.length; i++) { System.out.println(newObjs[i]); } } }
|